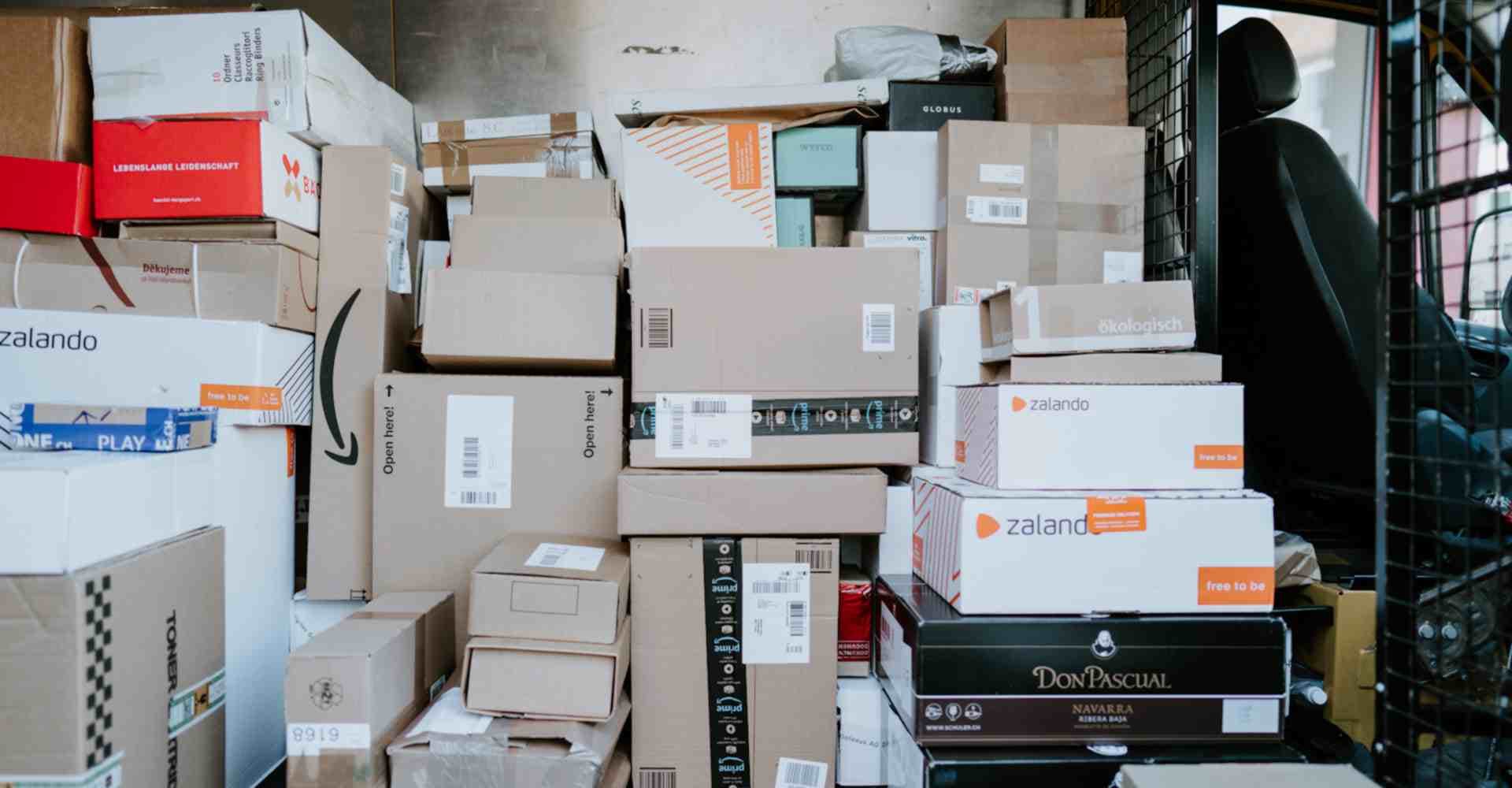
The top 8 npm packages I use for every project
Anime.js
Anime.js is a great JavaScript animation library that I use for just about every animation that can't be done quickly with CSS animations. It's also a super lightweight package. This library is great for so many different kinds of animations including CSS animations and transitions, JavaScript style animations, SVG morphing animations, and more.
This package is also available with npm and can be installed like this:
npm install animejs
#or
yarn add animejs
After installing the package you can import it into your JS files with this import, and follow the Documentation for how to make amazing animations.
import anime from "animejs";
If you would like to learn more about this package and how to use it I have written another article on the basics of animating with AnimeJS
Normalize.css
Normalize.css is just a CSS file that you include in your project to 'reset' the CSS of the browser so that your code works better across as many browsers and devices as possible.
npm install normalize.css
#or
yarn add normalize.css
To include this package in your project you can add it to your main CSS or SCSS/SASS file.
// This @import will change depending on where your main CSS is located.
@import "../../../node_modules/normalize.css/normalize.css";
If anyone knows a better way to get to the root directory of node_modules please let me know in the comments.
Prettier
If you haven't already started using Prettier then you need to right now. Prettier takes care of formatting your code to your preferences so you can make typos and mistakes without needing to fix them later.
Prettier can be set up in many different ways like formatting on file save and formatting on git commit, which is exactly what pretty-quick and Husky can do.
Pretty-quick and Husky
Pretty-quick is just Prettier but can be run as a hook when committing your changes. Husky is a package that runs code hooks from the package.json
file.
npm install pretty-quick husky
#or
yarn add pretty-quick husky
If you want to add this to your project just add this to your package.json
file.
"husky": {
"hooks": {
"pre-commit": "pretty-quick --staged"
}
}
'Git: husky > pre-commit (node v13.0.1)' error
If you are using Visual Studio Code and select a few lines of code the put into staging then just commit those few lines of code and you receive an error from prettier. I find that if you just ignore it and click cancel
then try to commit again it will work. I'm not sure why this happens but if you know, send me a message.
Browser sync
Browser sync is great for the development of a website because it lets you view a live version of the site and reloads the page when something in the code changes. It is also great for testing your site on different devices.
npm install -g browser-sync
#or
yarn global add browser-sync
For static sites, you can run a variation of this command.
browser-sync start --server --files "css/*.css"
And for dynamic sites running on a local server, you will have to run a variation of this command. For this command to work you will have to have already set up something like a Vagrant or Docker image.
browser-sync start --proxy "yourproject.dev" --files "css/*.css"
Eslinter
Eslint checks your JavaScript files against a set of rules that you can modify and tells you if your code is broken or missing anything that will break later. This includes things like missing semicolons and proper spacing.
There are too many different ways to set Eslinter up depending on your dev server, but if you are looking to add this to your Gridsome project check out the Documentation here.
Stylelint
Stylelint is the same as Eslint but for your stylesheets. This check to make sure you are spacing elements correctly and not missing semicolons.
There is no current way to add this to Gridsome.