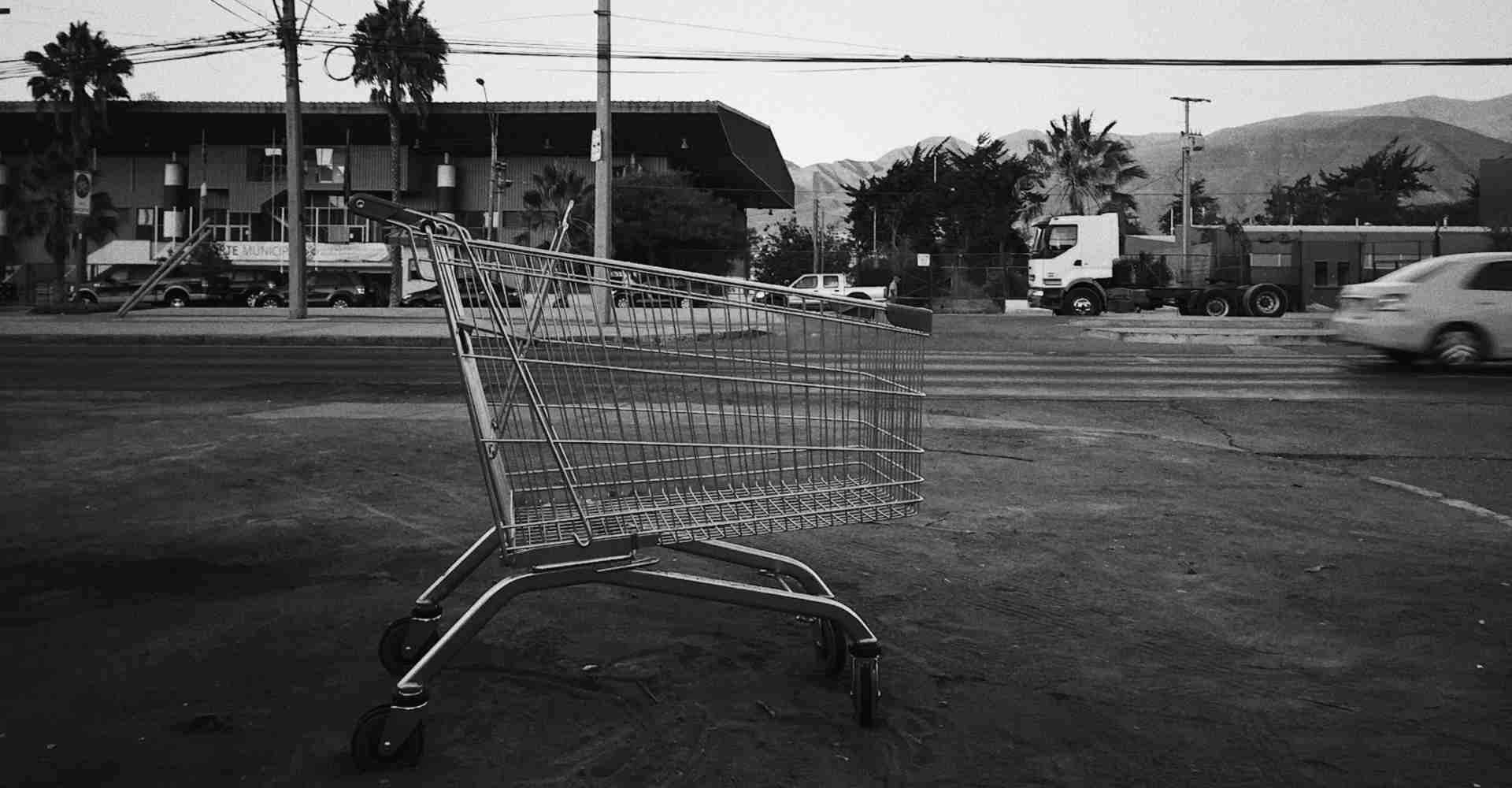
Making a shopping cart system with PHP 7.4 and MySQL
This code example is just a demo to show what is possible, and should not be used for actual e-commerce websites.
let's assume that you already have a product page and are displaying these products from a MySQL database, and now you want to make a cart system so a user can purchase more than one item at a time.
Product detail page and add to cart form
This page shows an individual item from the products page and will contain a form to add this item to the cart of the website. This form will go to an addtocart.php
file that will set the cookie for the cart. The form will look something like the below code.
The ID for this form would have been grabbed from a MySQL database.
print("
<form action='addtocart.php?id=$id' method='post'>
<label for='quantity'>Quantity: </label>
<input type='number' name='quantity' id='quantity' min='1' max='20' value='1'>
<input type='submit' name='add' id='add' value='Add to Cart'>
</form>
");
Adding to the cart
Now for the addtocart.php
file. For this page, you will want to connect your MySQL server to get the items from the database to add to the cart. You will also want to make sure that you tell PHP that you want to start using sessions with the session start function.
session_start();
Connecting to your MySQL database should look something like this.
$server = "localhost";
$username = "username";
$password = "123456789";
$database = "e-commerce";
$connection = mysqli_connect($server, $username, $password, $database);
/* And just in case something goes wrong */
if(!$connection) {
die("Connection Failed: " . mysqli_connect_error());
}
Once you have connected to the server we can add the item to the session. Because of the detail page form, the URL should look something like this example.com/addtocart.php?id=1
which means we can grab the ID of the item from the current URL.
Even though the detail page form has the method of post we can still use GET
to grab the id because we added it to the action of the form.
// Check if the add to cart button was clicked or if someone just wants to load this page. This POST method `add` is from the id of the add to cart button on the detail page.
if(isset($_POST['add']) && isset($_GET['id'])) {
$id = $_GET['id'];
// Check if there is a session for the cart set already, if not then set one.
if(!isset($_SESSION['shoppingcart'])) {
$_SESSION['shoppingcart'] = [];
}
$quantity = $_POST['quantity'];
$item = [
"id" => $id,
"quantity" => $quantity
];
// Now add a new item to the cart
array_push($_SESSION['shoppingcart'], $item);
// Once added let's take the user to the cart page
header("Location: cart.php");
} else {
// If the add to cart button was not clicked then go back to the products page.
header("Location: products.php");
}
Cart page and checkout
This page will display all of the items in the cart, have a checkout section, and remove all of the cart items.
Cart item removal
To remove all of the items from the cart let's start with the function that will check if the customer would like to do so and add the form that will trigger this action.
if(isset($_GET['reset'])) {
session_start();
unset($_SESSION['shoppingcart']);
session_destroy();
}
// Start the session for the rest of the page after destroying it.
session_start();
For the form that will remove all of the items, it would look like this. This form will simply reload this file and add ?reset=RESET
to the URL activating the PHP script above to remove the items.
<form action="#" method="GET">
<label for="reset">Reset cart: </label>
<input type="submit" value="RESET" name="reset" id="reset" />
</form>
Cart items display
Now to display all of the items that are in the cart session and the MySQL database.
// First check if the session is set
is(isset($_SESSION['shoppingcart']) && count($_SESSION['shoppingcart']) != 0) {
$list = $_SESSION['shoppingcart'];
foreach($list as $item) {
$id = $item['id'];
// The MySQL database query for the items in the cart.
$query = "SELECT * FROM products WHERE id='$id'";
// The connection variable is taken from the top of the page when we connected to the MySQL database
$result = mysqli_query($connection, $query);
// If only one item in the database has this id then proceed
if(mysqli_num_rows($result) == 1) {
$row = mysqli_fetch_array($result);
$id = $row['id'];
$name = $row['name'];
$price = $row['price'];
$quantity = $item['quantity'];
// Now let's print out this item to HTML
print(
"<div class='product-card'>
<a href='product-detail.php?id=$id'>
<h3>$name</h3>
<h4>$$price CAD</h4>
<h4>Quantity: $quantity</h4>
</a>
</div>");
}
}
} else {
print("<h2>You don't have any items in your shopping cart.</h2>");
}