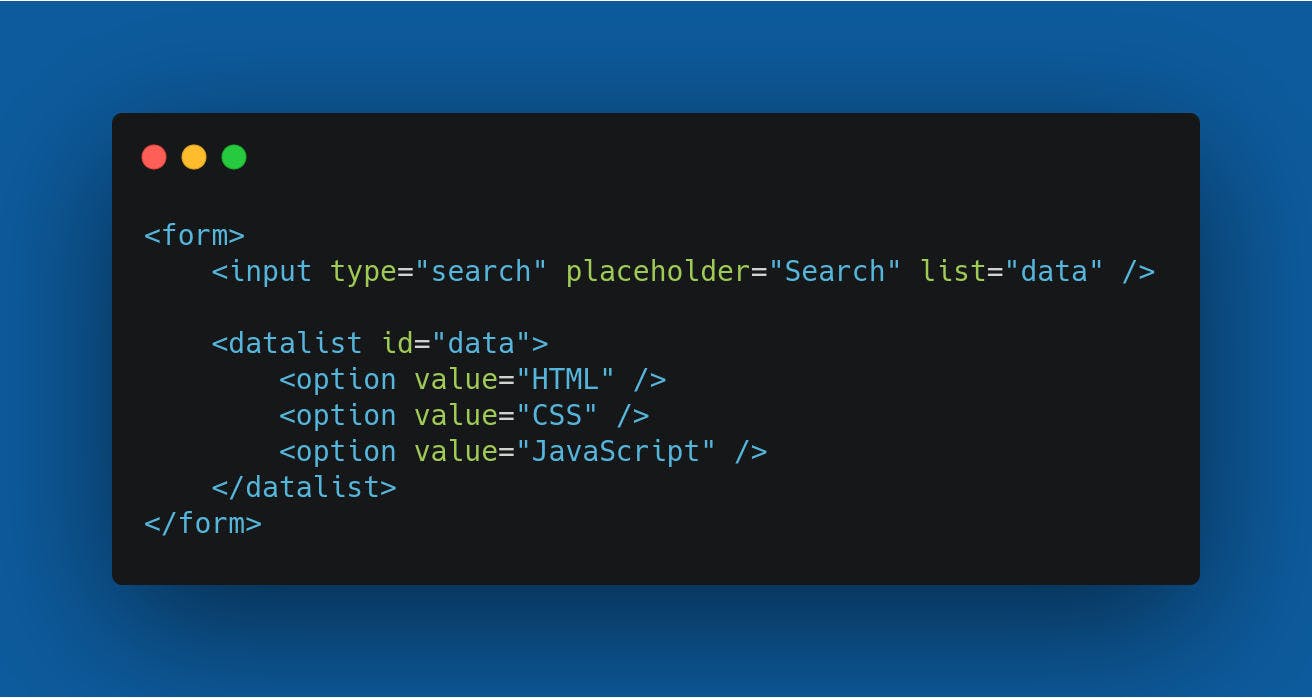
How to make a html search datalist for Vue
What do I mean by search suggestions
An example where I have to use a datalist is on the search page. Another example of this in action would look like this:
Adding the datalist to an input
To add a datalist to a text input you will need to add list="id"
to your text input. For this, to work the list attribute needs to be set to the id of the datalist element.
<input type="search" placeholder="Search" list="data" />
Creating the datalist element
The datalist element is like the select element.
<datalist id="data">
<option value="HTML" />
<option value="CSS" />
<option value="JavaScript" />
</datalist>
Implementing Vue
let us say you have a search form component using the above datalist and search input.
<form>
<input type="search" placeholder="Search" list="data" />
<datalist id="data">
<option value="HTML" />
<option value="CSS" />
<option value="JavaScript" />
</datalist>
</form>
Let's say that you have an array of search values that you would like to display in this datalist, for example, this array:
export default {
data() {
return {
list: ["HTML", "CSS", "JavaScript"]
};
}
};
<form>
<input type="search" placeholder="Search" list="data" />
<datalist id="data">
<option v-for="item in list" :key="item" :value="item" />
</datalist>
</form>
Adding Gridsome
So you are using Gridsome (like me). Let's say that you would like your post titles to show in this data list, you will need a page-query
and use that instead of the data array.
<page-query>
query Search {
allPost {
edges {
node {
title
}
}
}
}
</page-query>
Now for the new datalist.
<form>
<input type="search" placeholder="Search" list="data" />
<datalist id="data">
<option v-for="{ item } in $page.allPost.edges" :key="node.title" :value="node.title" />
</datalist>
</form>